trOnGAtE
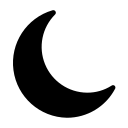
Generating Tokens (JavaScript Friendly Version)
Now, with the assumption that your database is ready for action, let's explore how to generate security tokens with Trongate. The instructions here have been designed to be JavaScript friendly.
Testing Token Management
If you are in 'dev' mode, you should be able to access Trongate's API Manager. This is a feature that will help you to easily test your token management. The URL for handling 'trongate_tokens' is,
http://localhost/your_app/api/explorer/trongate_tokens

Inside your 'modules' folder, there's a module named 'trongate_tokens'. This module contains a controller file with a PHP class named, 'Trongate_tokens'. This class can be used to generate security tokens.
The 'Generate Token' API Endpoint
Trongate apps contain an API endpoint that can be used to generate tokens via HTTP POST requests. The URL for generating tokens is your base URL followed by trongate_tokens/generate.
function generate() {
die(); //disable this endpoint!
/*
generate token by POST (for devs who like JavaScript)
$posted data may contain:
user_id ~ int(11) : required
expiry_date ~ int(10) : optional
*/
if ($_SERVER["REQUEST_METHOD"] !== 'POST') {
http_response_code(403);
echo 'Forbidden';
die();
} else {
//fetch posted data
$posted_data = file_get_contents('php://input');
$input = (array) json_decode($posted_data);
$data = $this->_pre_token_validation($input);
}
$token = $this->_generate_token($data);
http_response_code(200);
echo $token;
}
Required Parameters
In order to generate a token, you will be required to post a 'user_id' value. This should be a numeric value that represents the ID, on the trongate_users table, that corresponds with the user who is requesting a new token.
You may also, if you wish, submit an optional 'expiry_date'. The expiry_date should be a Unix timestamp representing the date and time when token should expire.
<?php
$expiry_date = time() + (86400*3);
?>
The screenshot below show an example of Trongate's API Manager being used to test token generation where a user_id and expiry_date have been submitted. As you can see (near the top right hand side) the server has responded with a '200' status code and a token has been issued.

Token Generation For Live Apps
Once your Trongate app is in a live environment - in other words, your ENV value (as declared in config.php) is no longer set to 'dev' - then token generation becomes a little more strict.
Clearly, it would be a security risk if we allowed anyone to generate tokens simply by submitting a user_id to a known endpoint.
Therefore, in live environments you will be required to add your own additional validation code inside the _pre_token_validation() method, as declared in Trongate_tokens.php. By default, this method contains the following code:
function _pre_token_validation($input) {
if (ENV !== 'dev') {
//add your own validation code here!
echo 'Forbidden (no validation tests available)';
http_response_code(403);
die();
}
if (!isset($input['user_id'])) {
http_response_code(400);
echo 'No user_id submitted!';
die();
} elseif(!is_numeric($input['user_id'])) {
http_response_code(400);
echo 'Non-numeric user_id submitted!';
die();
}
return $input;
}
Your job, therefore, would be to add your own custom validation tests inside the first IF statement of the _pre_token_validation() method. The precise code that will make up your validation tests is a decision for you, the developer, and falls outwith the scope of this documentation. However, for clarity, here is the method again - this time a few lines removed and comments indicating where you should add your own validation tests:
function _pre_token_validation($input) {
if (ENV !== 'dev') {
//add your own validation tests here
//for example, check posted username and password
}
if (!isset($input['user_id'])) {
http_response_code(400);
echo 'No user_id submitted!';
die();
} elseif(!is_numeric($input['user_id'])) {
http_response_code(400);
echo 'Non-numeric user_id submitted!';
die();
}
return $input;
}
$posted_data = file_get_contents('php://input');
$data = (array) json_decode($posted_data);
HELP & SUPPORT
If you have a question or a comment relating to anything you've see here, please goto the Help Bar.